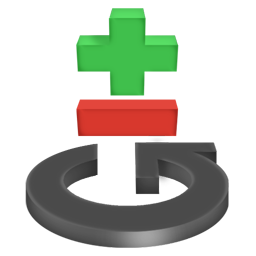
Git is a distributed Version control and Source code management system. It was designed by Linus Torvalds ( The Brain behind Linux Kernel ). It is used widely in management of Source code of a project.
Extremely helpful when an application is being developed by more than one developer. Each developer will be updated about all the changes carried out to the source code by other developers.
This article takes a simplified approach of explaining the basic commands used in Git .
Lets get started.
Git Configuring :
Before you start using Git , you need to configure the Git such as setting up Name , Email , Key etc. Use
$ git config --global user.name "My Name"
$ git config --global user.email "user@domain.com"
Git Initialization :
To work with Git , you need to initialize the repository.
Just move into the directory you want to initialize and use.
$ git init
Git Staging :
After initialization of the repository (directory) , you need to stage the files. You can consider Git to have 3 stages : Unstaged , Staged and Committed. Stage the files using (If you want to stage all the files in the directory) :
$ git add *
else if for staging individual files use :
$ git add file-name
else for staging multiple files use :
$ git add file-name1 file-name2 filename3
Git Committing :
After staging of the files, you need to commit them. Committing the files means you are ready to push the files to either a local or a remote repository. To commit the files , you should always first stage those files. For committing use (-m option is used to put some commit message) :
$ git commit -m "Commit Message"
Git Pushing to a Remote Repository :
Usually after the committing the files, you would like to push it to some remote repository for other developers to collaborate. To set up a remote repository you have add that remote repository. ( Usually we use either github or bitbucket ). Create an account in either of those sites and create a repository. Then use (Test is the name of the remote repository):
$ git remote add origin https://github.com/rShetty/Test.git
$ git push -u origin master
After adding the remote repository use the "git push" command to push your committed files to the remote repository. That is all you have to do for putting your code to a remote repository.
Git Checking Status :
At any time while using Git,If you need to check the status of the files. You can use :
$ git status
This command gives you the status of your files like ( Staged , Not Staged or Committed or Modified etc ).
Git Cloning :
Git cloning is making copying of the remote repository onto your local machine. To clone a Remote repository use :
$ git clone git@github.com:username/test.git
Git Branching :
Whenever you initialize a git repository, a Master Branch is created for you to work on. In some cases, where you want to try some stuff on your code and don't want to disturb your code in Master branch, you can create branches using
$ git branch branch-name
and can shift to that branch using :
$ git checkout branch-name
The above two commands can be collapsed into a single one , Using this you will create a new branch and shift to it.
$ git checkout -b branch-name
Git Merging :
So here you have tried some cool new feature in a branch and it does work fine and you want to integrate that feature into the main branch (Master). To Integrate the changes you need to merge the branches as :
$ git merge
Git Pulling and Fetching :
You use :
$ git fetch origin
When you want to fetch all the objects which are not there in your local repository.
and
$ git pull origin
To fetch the files from the remote repository and merging it to the local one.
Git Logging :
Git Log is used to check the logs of your commits, changes etc you made over a period of time. It provides complete information of various commits , branches and the person responsible for the same.
$ git log
or
$ git log -n 10
To see the last 10 git log commits.
Git Tagging :
Provides a Tag to commit you made with a simple Human readable handle.
$ git tag -a v1.0 -m 'Version 1.0'
Git Undoing Changes :
A lot of times we stage files which we should not have or we commit wrong files. To undo these Human errors, Git provides flexible and easy commands.
Use : If you want to unstage a file, which you have staged by mistake use the above command.
$ git reset HEAD file-name
Use : If you want to change your last commit.
$ git commit --amend
Use: If you want to undo all the changes you made to a file (Use this carefully)
$ git checkout -- file-name
GitK / GitX :
These are the UI based Git clients. GitK comes for Windows and Linux and GitX is for MAC. Install it and use as :
$ gitk
$ gitx
That's all , These commands will keep you up running with Git.
Git is a very Powerful and efficient tool . Use it Wisely .
Explore some lesser known git commands here :
Explore some lesser known git commands here :
For any Doubts/ Clarifications/Feedback write to me @
or Connect me on Twitter @rBharshetty
3 comments:
Rather than specifying the commit message on the command line with -m, it's probably better to write a more complete commit messages by leaving off the -m and using an editor to put together a message in the following format:
1. A one line summary of the changes for "shortlog"
2. A blank line to separate
3. A more detailed description to document the exact nature of the changes.
This makes your "git log" output much more usable and friendly. Linus (who wrote git) talks about how to write a good commit message in various places but the first one I found on Google was here:
https://github.com/torvalds/subsurface/blob/master/README
How about git rebase?
Git Branching and Rebasing
http://git-scm.com/book/en/Git-Branching-Rebasing
Post a Comment